Sources
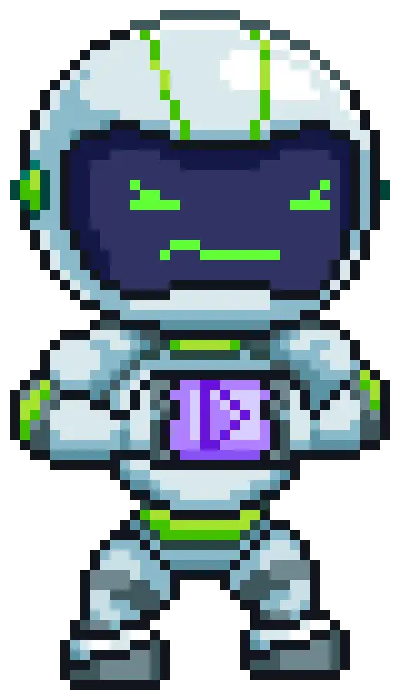
Introduction
Sources are File Objects with a kind
of video
, audio
, or image
.
They represent the files in your project that contain the actual content.
Media objects can contain one or more Sources.
Usage
At it's simplest, a source is what you'd pass to an <img>
, <video>
, or <audio>
tag's src
attribute.
In production projects, you'll usually want to provide multiple sources for a media object.
For example, you might want to generate a poster image for the video, which can be used to display a thumbnail or preview before playback begins.
You might then want to provide a version of the video in the more efficient WEBM format for browsers that support it, and fallback to your MP4 version for browsers that don't.
See Media for more examples.
Media
Kinds
Sources can be of the following kinds:
video
audio
image
Video
Commonly used in <video>
tags or as input for mobile, TV, and other video media players.
Codecs & Containers
Uploaded or ingested files can contain:
Property | Values |
---|---|
Video Stream Codec | h264/avc , h265/hevc , vp8 , vp9 , av1 |
Audio Stream Codec | aac , opus , mp3 , flac , vorbis |
Media Container | mp4 , webm , mov , avi , mkv |
Tasks can output:
Property | Values |
---|---|
Video Stream Codec | h264/avc , vp9 |
Audio Stream Codec | aac , opus |
Media Container | mp4 , webm |
If you require hevc
, av1
, or any other codecs or containers, please contact us.
Resolution
Uploaded or ingested files can be:
- upto
4096px
on the longest edge - upto
16MP
in total pixels
Tasks have the same default limits. 1
Duration
Uploaded or ingested files can be:
- upto
4 hours
in duration
Tasks have the same default limits. 1
Filesize
Uploaded or ingested files can be:
- upto
4TB
in size
Tasks have the same default limits. 1
New Video
See the Video Tasks section for more information about creating new video sources.
Video Tasks
Image
Commonly used in <img>
or <picture>
tags.
Formats
Uploaded or ingested files can be:
Property | Values |
---|---|
Image Format | jpeg , png , webp , gif , hevc /heic , avif |
Tasks can output:
Property | Values |
---|---|
Image Format | jpeg , png , webp , gif , avif |
If you require svg
support, or need to support files in any other format, please contact us for early access.
Resolution
Uploaded or ingested files can be:
- upto
8192px
on the longest edge - upto
32MP
in total pixels
Tasks have the same default limits. 1
HDR
We support HDR images in formats that allow it:
heic
/heif
with HEVC codecavif
with AV1 codecwebp
with VP8 codec
Animation
We support animated gif
, webp
, and avif
images.
Transparency
We support transparency in png
, webp
, avif
, and gif
images with an alpha channel.
Filesize
Uploaded or ingested files can be:
- upto
4TB
in size
New Image
See the Image Tasks section for more information about creating new image sources.
Image Tasks
Audio
Commonly used in <audio>
tags.
Formats
Uploaded or ingested files can be:
Property | Values |
---|---|
Audio Stream Codec | aac , opus , mp3 , flac , vorbis |
Media Container | mp3 , m4a , wav , ogg |
Tasks can output:
Property | Values |
---|---|
Audio Stream Codec | aac , opus , mp3 |
Media Container | mp3 , ogg , wav |
If you require flac
or any other audio codec, please contact us for early access.
Duration
Uploaded or ingested files can be:
- upto
4 hours
in duration
Tasks have the same default limits. 1
Filesize
Uploaded or ingested files can be:
- upto
4TB
in size
Tasks have the same default limits. 1
New Audio
See the Audio Tasks section for more information about creating new audio sources.